Here, you will learn about how a thread is accessed by using the hash table in Java.
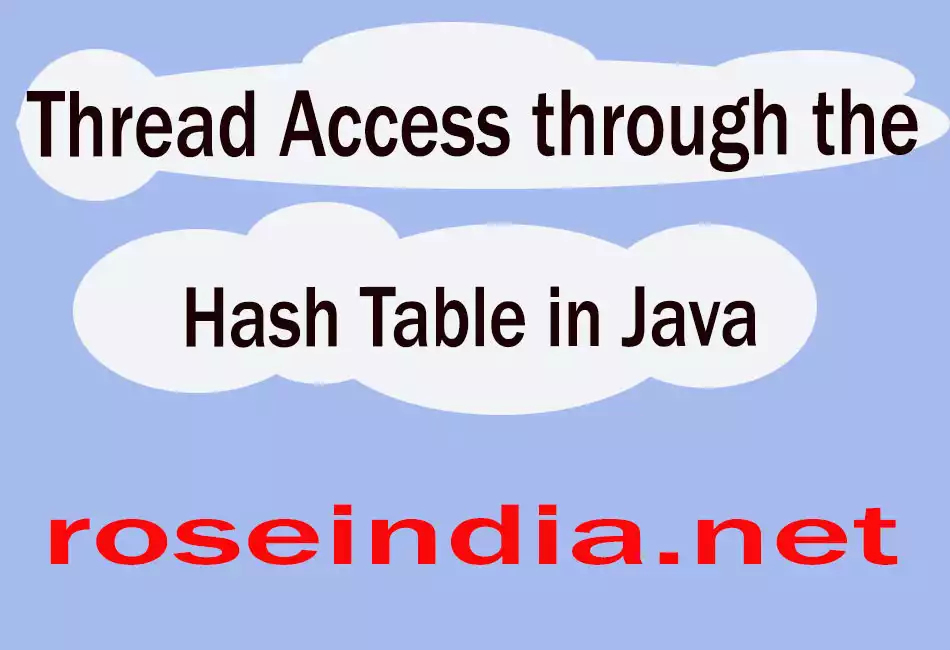
Thread Access through the Hash Table in Java

Here, you will learn about how a thread is accessed by
using the hash table in Java.
Here is the code of the program:
import java.util.*;
public class ThreadedAccess implements Runnable{
static int ThreadCount = 0;
public void run(){
String s = "This is thread " + ThreadCount++;
Vector<String> v = new Vector<String>();
v.addElement(s);
v.addElement(s);
try{
Thread.sleep(2000);
}
catch(Exception e){}
v.addElement(s);
System.out.println(v);
}
public static void main(String[] args){
try{
for(int i = 0; i < 5; i++){
(new Thread(new ThreadedAccess())).start();
try{
Thread.sleep(200);
}
catch(Exception e){}
}
}
catch(Exception e){
e.printStackTrace();
}
}
private static ThreadLocal<Vector> vectors =
new ThreadLocal<Vector>();
public static Vector getVector(){
Vector v = (Vector) vectors.get();
if (v == null){
v = new Vector();
vectors.set(v);
}
return v;
}
private static Hashtable<Thread,Vector> hvectors =
new Hashtable<Thread,Vector>();
public static Vector getVectorPriorToJDK12(){
Vector v = (Vector) hvectors.get(Thread.currentThread());
if(v == null){
v = new Vector();
hvectors.put(Thread.currentThread(), v);
}
return v;
}
}
|
Download this example.