A message-driven bean is a new type of enterprise bean defined in the EJB 2.0 specification as an asynchronous message consumer. The bean is invoked by the container as the result of the arrival of a Java Message Service (JMS) message. The listener manager passes the message via a listener port to the bean. Message-driven beans are stateless, server-sided, and not distributed components. They do not have EJBObject and EJBHome references. They are invoked by the container as soon as an asynchronous messages is delivered from JMS. Once the message arrived on the queue, the EJB cannot wait to receive a JMS message.
Message-driven beans are stateless, server-side, transaction-aware components that process asynchronous messages delivered via the Java Message Service (JMS). Asynchronous messaging allows applications to communicate by exchanging messages in a way that leaves senders independent of receivers. That is, the sender sends its message and does not have to wait for the receiver to receive or process that message. Message-driven beans serve as router processes that operate on inbound enterprise messages from a JMS provider. You might use a message-driven bean to integrate an EJB-based system with a legacy system or to enable business-to-business interactions. The message-driven bean's sole responsibility is processing messages, because its container automatically manages the component's entire environment.
To a client, a message-driven bean is a JMS consumer that implements some business logic running on the server. A client accesses a message-driven bean by sending messages to the JMS destination for which the bean class is the MessageListener. The destination is either a Queue or a Topic.
Message-driven beans subscribe to specific message destination, which is a ListenerPort mapped to a queue or topic name. The ability to concurrently process messages makes message-driven beans extremely powerful enterprise beans. An EJB container can process thousands of messages from various applications concurrently by leveraging the number of bean instances. There is no guarantee about the exact order delivered to the bean instances. The bean should be prepared to handle messages that are out of sequence.
You can use the Create an Enterprise Bean wizard to create a message-driven bean.
To create a message-drive bean, you must first have an EJB 2.0 project defined. Message-driven beans are only supported for EJB 2.0 projects.
To create a message-driven bean:
In the J2EE perspective, click File > New > Enterprise Bean. The Create an Enterprise Bean wizard appears.
Select the EJB project that you want to add the bean to.
Select Message-driven bean from the radio button list.
In the Bean name field, type in the name that you want to assign to the enterprise bean. When you type the Bean name, the Bean class field is updated. For example, if you type "ReservationProcessor" in the Bean name field, the Bean class field is updated with "ReservationProcessorBean". You may choose to override the default entries. The fields are on the next page of the wizard. The Bean name should begin with a capital letter.
NOTE: You can use Unicode for the bean's name, but Unicode characters are not supported for enterprise bean packages/classes associated with enterprise beans.
Click Next to specify additional information for the new bean, or click Finish to take the defaults for the new message-driven bean.
Select a transaction type for the message-driven bean. You can select from the following options:
Container - If you select this option, the container manages transactions on behalf on of the bean.
Bean - If you select this option, the message bean manages its own transactions, and you can also select an Acknowledge mode. The Acknowledge Mode is how the session acknowledges any messages it receives. Acknowledge mode: AutoAcknowledge or DupsOkAcknowledge.
In the Message driven destination section, select a Destination Type. If you select a destination type of topic, then you can also select a value for Durability. The durability property defines whether a JMS topic subscription is durable or non-durable.
If you set the value to durable, a subscriber registers a durable subscription with a unique identity that is retained by JMS. Subsequent subscriber objects with the same identity resume the subscription in the state it was left in by the earlier subscriber. If there is no active subscriber for a durable subscription, JMS retains the subscription's messages until they are received by the subscription or until they expire.
If you set the value to nondurable, the subscriptions last for the lifetime of their subscriber object. This means that a client sees the messages published on a topic only while its subscriber is active. If the subscriber is not active, the client is missing messages published on its topic.
Bean supertype: This option will only display other message-driven beans in the EJB project.
Bean class: Type or select the desired bean class.
Type a Message selector. The JMS message selector to be used to determine which messages the message bean receives.
Type a ListenerPort name. A listener port is used to simplify administration of the association between a connection factory, destination, and deployed message-driven bean. Each listener port is used with a message-driven bean to automatically receive messages from an associated JMS destination.
Click Next to specify additional information for the new bean, or click Finish to take the defaults for the new message-driven bean.
In the Bean superclass field, type or select the desired class.
Click Finish. The new enterprise bean appears under the selected EJB project.
Add the following code to the com.titan.reservationprocessor.ReservationProcessorBean:
private javax.jms.MapMessage msg = null;
public void onMessage(javax.jms.Message inMessage) { try { if (inMessage instanceof javax.jms.MapMessage) { msg = (javax.jms.MapMessage) inMessage; String cust = msg.getString("name"); String prod = msg.getString("cruise"); System.out.println("ReservationProcessor: Customer: " + cust); System.out.println("ReservationProcessor: Cruise: " + prod); } else { System.out.println("ReservationProcessor: Wrong type."); } } catch (javax.jms.JMSException e) { e.printStackTrace(); fMessageDrivenCtx.setRollbackOnly(); } catch (Throwable te) { te.printStackTrace(); } }
Define Listener Port for MDB
Right-click the titan project in the J2EE Hierarchy view and select Open With > Deployment Descriptor Editor. On the Beans page, select ReservationProcessor and view the associated values in the editor.
In the field ListenerPort name type LP1. 0
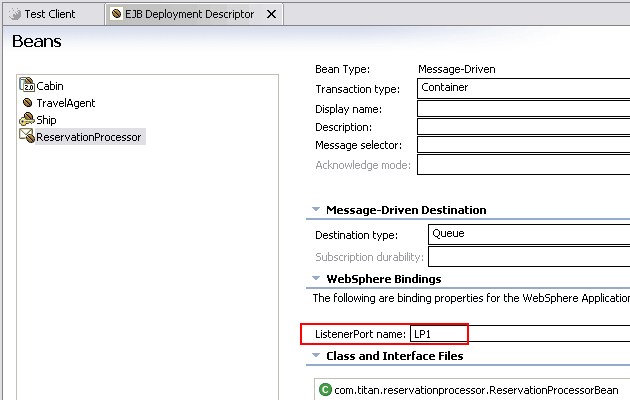