In this section, you will learn how to make a file to be read into a byte array. Here we have used inputStream constructor to take file as a parameter.
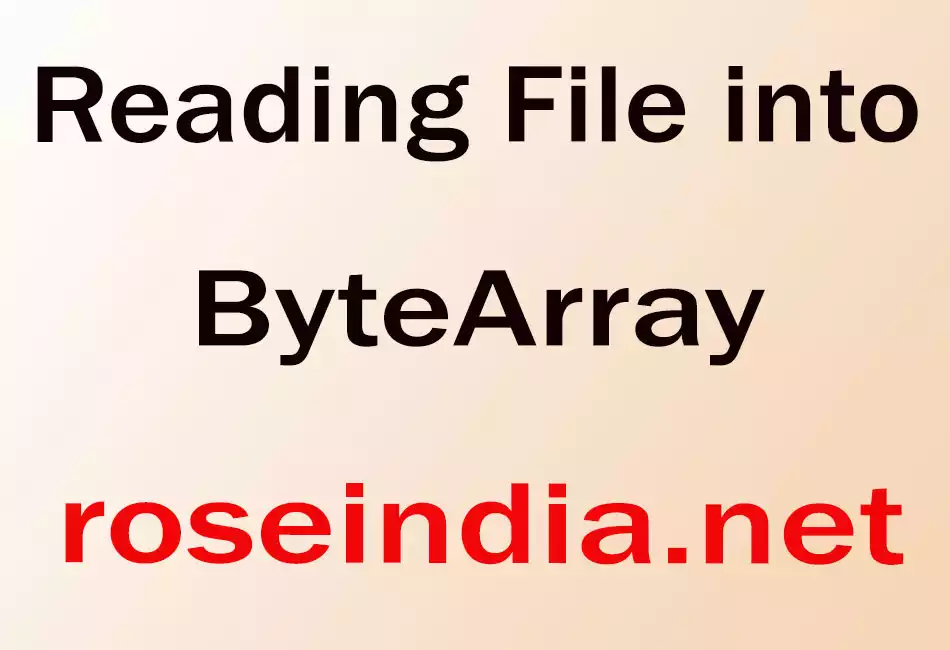
Reading File into ByteArray

In this section, you will learn how to make a file to
be read into a byte array. Here we have used inputStream constructor to take
file as a parameter.
Description of code:
In this program we have taken a file which is to be read into an array of byte.
We have used file.length(); method to take the length of the file. And if
the file is very large it will give the message that the file is too large. Then
we taken two variables offset and numRead so that the file could
be read from zero. Then the file will be converted to the bytes with the help of
BytesFromFile(new File("test.txt"));.
Here is the code of program:
import java.io.File;
import java.io.InputStream;
import java.io.FileInputStream;
import java.io.IOException;
public class FileReadInByteArray {
public static byte[] BytesFromFile(File file) throws IOException {
InputStream is = new FileInputStream(file);
long length = file.length();
if (length > Integer.MAX_VALUE) {
System.out.println("Sorry! Your given file is too large.");
System.exit(0);
}
byte[] bytes = new byte[(int)length];
int offset = 0;
int numRead = 0;
while (offset < bytes.length && (numRead=is.read(bytes,
offset, bytes.length-offset)) >= 0) {
offset += numRead;
}
if (offset < bytes.length) {
throw new IOException("Could not completely read file "
+ file.getName());
}
is.close();
return bytes;
}
public static void main(String[] args) {
byte[] byteArray = null;
try {
byteArray = BytesFromFile(new File("test.txt"));
} catch (IOException e) {
e.printStackTrace();
}
if (byteArray != null) {
System.out.println("Your File Content: ");
for (int i=0; i < byteArray.length; i++) {
System.out.print((char)byteArray[i]);
}
}
}
}
|
|
Output of the program:
C:\chandan>javac FileReadInByteArray.java
C:\chandan>java FileReadInByteArray
Your File Content:
Chandan Kumar Verma
C:\chandan> |
Download this example.