This section illustrates you how to read and write from/to a serialized file through the hash table in Java.
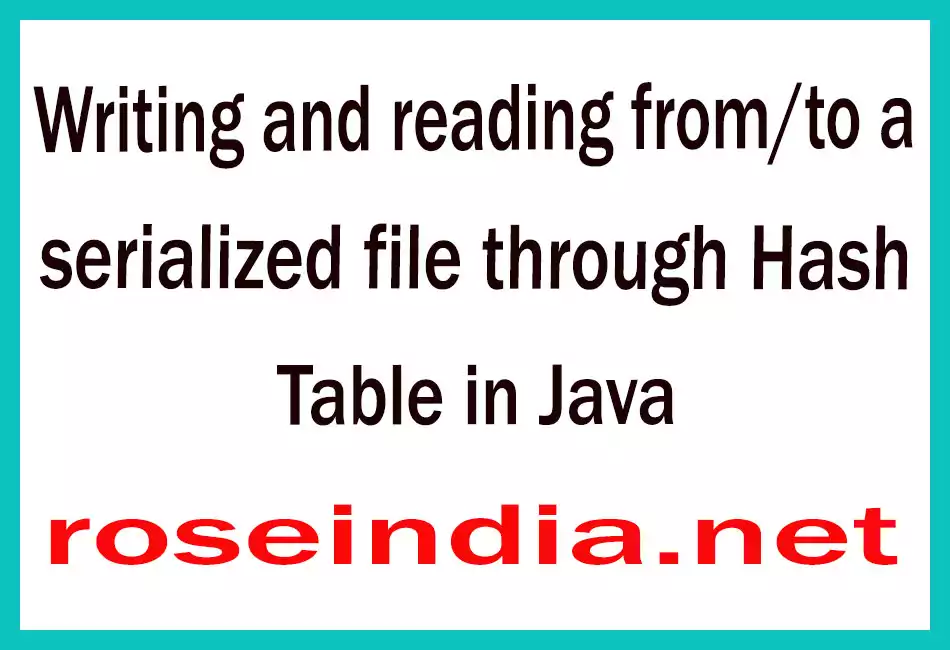
Writing and reading from/to a serialized file through
Hash Table in Java

This section illustrates you how to read and write
from/to a serialized file through the hash table in Java. This section provides
an example with the complete code of the program. Following program has the
facility if the specified serialized file does not exist the the program creates
the serialized file otherwise. This program first write to the specified
serialized file if it exists otherwise read all contents by de-serializing the
file.
Code Description:
FileOutputStream fileOut = new FileOutputStream("HTExample.ser"):
Above code creates an object "fileOut" of the FileOutputStream
with it's constructor which takes a file name which contains ".ser"
extension that determines for the serialized file either which has to be created
or written with the specified value in a hash table in the program.
Here is the code of the program:
import java.util.*;
import java.io.*;
public class SimpleSerialization{
private static void doSave(){
System.out.println();
System.out.println("+------------------------------+");
System.out.println("| doSave Method |");
System.out.println("+------------------------------+");
System.out.println();
Hashtable<String,Object> h = new Hashtable<String,Object>();
h.put("string", "Oracle / Java Programming");
h.put("int", new Integer(36));
h.put("double", new Double(Math.PI));
try{
System.out.println("Creating File/Object output stream...");
FileOutputStream fileOut = new FileOutputStream("HTExample.ser");
ObjectOutputStream out = new ObjectOutputStream(fileOut);
System.out.println("Writing Hashtable Object...");
out.writeObject(h);
System.out.println("Closing all output streams...\n");
out.close();
fileOut.close();
}
catch(FileNotFoundException e){
e.printStackTrace();
}
catch(IOException e){
e.printStackTrace();
}
}
private static void doLoad(){
System.out.println();
System.out.println("+------------------------------+");
System.out.println("| doLoad Method |");
System.out.println("+------------------------------+");
System.out.println();
Hashtable h = null;
try{
System.out.println("Creating File/Object input stream...");
FileInputStream fileIn = new FileInputStream("HTExample.ser");
ObjectInputStream in = new ObjectInputStream(fileIn);
System.out.println("Loading Hashtable Object...");
h = (Hashtable)in.readObject();
System.out.println("Closing all input streams...\n");
in.close();
fileIn.close();
}
catch(ClassNotFoundException e){
e.printStackTrace();
}
catch(FileNotFoundException e){
e.printStackTrace();
}
catch(IOException e){
e.printStackTrace();
}
System.out.println("Printing out loaded elements...");
for(Enumeration e = h.keys(); e.hasMoreElements();){
Object obj = e.nextElement();
System.out.println(" - Element(" + obj + ") = " + h.get(obj));
}
System.out.println();
}
public static void main(String[] args){
doSave();
doLoad();
}
}
|
Download this example.