Show Addition, Subtraction, Intersection, Exclusive OR on one
Frame

This section illustrates you how to show Addition, Subtraction,
Intersection, ExclusiveOR on one frame.
In this section, we are providing you an example which performs all the functions on one
frame. Two classes Rectangle2D and Ellipse2D are used which
provides rectangle and circle.
A button panel is created containing the buttons. The ActionListener
class is called to perform all the actions on these buttons.
The a.area(a2) add the areas of two shapes. The a. area(a2)
subtract the area of a1 from a2. The a.intersect(a2) shows the
common area between the two shapes. The a.exclusiveOr(a2) shows the
uncommon area between the two shapes.
Here is the code of CalculateArea.java
import java.awt.*;
import javax.swing.*;
import java.awt.event.*;
import java.awt.geom.*;
public class CalculateArea {
public static void main(String[] args) {
JFrame frame = new AreaFrame();
frame.setVisible(true);
}
}
class AreaFrame extends JFrame implements ActionListener {
public AreaFrame() {
setTitle("Study the various functions");
setSize(450, 350);
Container contentPane = getContentPane();
canvas = new AreaPanel();
contentPane.add(canvas, "Center");
JPanel buttonPanel = new JPanel();
ButtonGroup group = new ButtonGroup();
button1 = new JButton("Add");
buttonPanel.add(button1);
group.add(button1);
button1.addActionListener(this);
button2 = new JButton("Subtract");
buttonPanel.add(button2);
group.add(button2);
button2.addActionListener(this);
button3 = new JButton("Intersect");
buttonPanel.add(button3);
group.add(button3);
button3.addActionListener(this);
button4 = new JButton("Exclusive Or");
buttonPanel.add(button4);
group.add(button4);
button4.addActionListener(this);
contentPane.add(buttonPanel, "South");
}
public void actionPerformed(ActionEvent event) {
Object source = event.getSource();
if (source == button1)
canvas.addAreas();
else if (source == button2)
canvas.subtractAreas();
else if (source == button3)
canvas.intersectAreas();
else if (source == button4)
canvas.exclusiveOrAreas();
}
private AreaPanel canvas;
private JButton button1;
private JButton button2;
private JButton button3;
private JButton button4;
}
class AreaPanel extends JPanel {
public AreaPanel() {
a1 = new Area(new Ellipse2D.Double(120, 120, 150, 120));
a2 = new Area(new Ellipse2D.Double(150, 150, 150, 120));
addAreas();
}
public void paintComponent(Graphics graphics) {
super.paintComponent(graphics);
Graphics2D graphics2D = (Graphics2D) graphics;
graphics2D.draw(a1);
graphics2D.draw(a2);
graphics2D.fill(a);
}
public void addAreas() {
a = new Area();
a.add(a1);
a.add(a2);
repaint();
}
public void subtractAreas() {
a = new Area();
a.add(a1);
a.subtract(a2);
repaint();
}
public void intersectAreas() {
a = new Area();
a.add(a1);
a.intersect(a2);
repaint();
}
public void exclusiveOrAreas() {
a = new Area();
a.add(a1);
a.exclusiveOr(a2);
repaint();
}
private Area a;
private Area a1;
private Area a2;
}
|
Output will be displayed as:
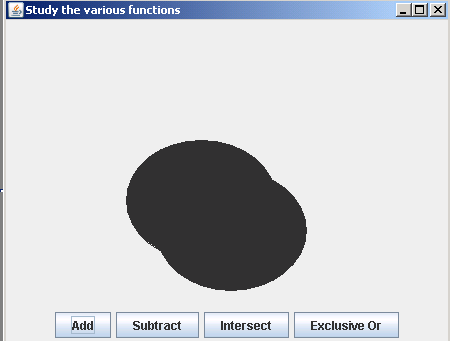
Download Source Code