In this section, we have developed an application to create xml file from flat file and data insert into database in Java.
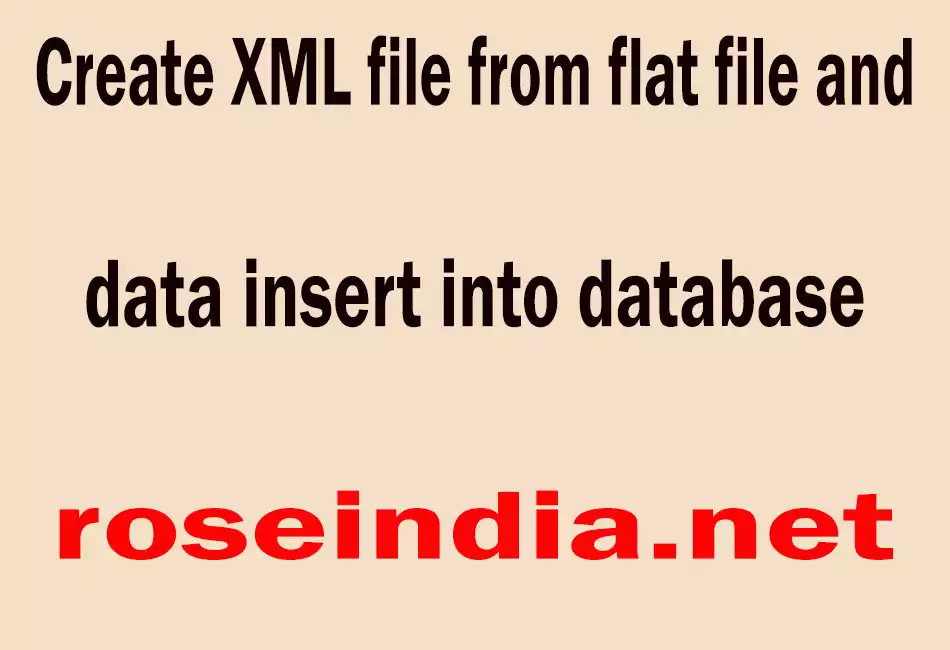
Create XML file from flat file and data insert into database

In this section, we have developed an application to
create xml file from flat file and data insert into database in Java.
Two files are used "FlatFileXml.java"
and "flatfile.txt" in
the code given below.
Flat file: A flat file is a
simple plain text file. It consists of one record per line.
The fields can be separated by delimiters, e.g. commas,
or have a fixed length. It
is a static document, spreadsheet, or textual record that typically contains data that is not structurally related.
Brief description of the flow of application :
1). Create a flat file "flatfile.txt".
2). Create a file "FlatFileXml.java"
used to create an XML and data insert into the database.
Step:1 Create a "flatfile" table in Database .
create table `flatfile` (
`id` int ,
`title` varchar (250),
`url` varchar (250)
)
|
Step:2 Create a "FlatFileXml.java"
import java.io.*;
import java.sql.*;
import org.xml.sax.*;
import org.xml.sax.helpers.*;
import javax.xml.parsers.*;
import javax.xml.transform.*;
import javax.xml.transform.stream.*;
import javax.xml.transform.sax.*;
public class FlatFileXml {
String url = "jdbc:mysql://localhost:3306/";
String dbName = "userdetails";
String driver = "com.mysql.jdbc.Driver";
String userName = "root";
String password = "root";
Statement stmt = null;
Connection con;
int val;
BufferedReader br;
StreamResult sr;
TransformerHandler tfh;
AttributesImpl ai;
public static void main(String args[]) throws Exception{
try
{
new FlatFileXml().datamain();
System.out.println("You are Successfully create XML and
data insert into the database.");
}
catch (Exception e)
{
System.out.println(e.getMessage());
}
}
public void datamain () {
try {
br = new BufferedReader(new FileReader("flatfile.txt"));
sr = new StreamResult("flatfile.xml");
xmlMain();
String str;
while ((str = br.readLine()) != null) {
doWork(str);
}
br.close();
xmlEnd();
}
catch (Exception e) { e.printStackTrace(); }
}
public void xmlMain() throws Exception {
SAXTransformerFactory tf = (SAXTransformerFactory)
SAXTransformerFactory.newInstance();
tfh = tf.newTransformerHandler();
Transformer serTf = tfh.getTransformer();
serTf.setOutputProperty(OutputKeys.ENCODING,"ISO-8859-1");
tfh.setResult(sr);
tfh.startDocument();
ai = new AttributesImpl();
}
public void doWork (String s) throws Exception{
String [] ar = s.split("\\-");
try{
Class.forName("com.mysql.jdbc.Driver");
con = DriverManager.getConnection(url+dbName,userName,password);
String queryString = "INSERT INTO flatfile ( title,url)
values('"+ar[0]+"','"+ar[1]+"')";
stmt=con.createStatement();
val = stmt.executeUpdate(queryString);
}
catch(Exception e)
{
System.out.println("e : " + e);
}
ai.clear();
tfh.startElement("","","TITLE",ai);
tfh.characters(ar[0].toCharArray(),0,ar[0].length());
tfh.endElement("","","TITLE");
tfh.startElement("","","URL",ai);
tfh.characters(ar[1].toCharArray(),0,ar[1].length());
tfh.endElement("","","URL");
}
public void xmlEnd() throws Exception{
tfh.endDocument(); }
}
|
Step:3 Create a flat file "flatfile.txt".
Java - http://www.roseindia.net
JSP - http://www.roseindia.net
SERVLET - http://www.roseindia.net
Struts - http://www.roseindia.net
|
Output:

After run Successfully Create an XML file "flatfile.xml"
Download the full Code