To reverse the image, an image is put into the folder where class is specified. The getDefaultToolkit() method of ToolKit class provides the default toolkit.
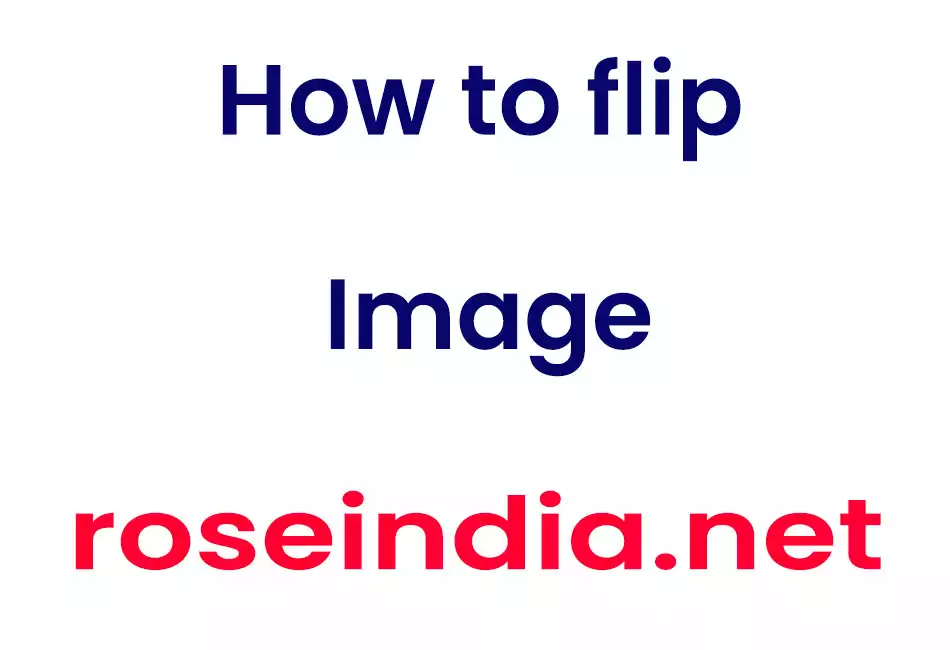
How to flip image

In this section , you will see how to flip image.
To reverse the image, an image is put into the folder where class is specified. The getDefaultToolkit() method of
ToolKit class provides the default toolkit. The method getImage() of
class ToolKit returns an image.
The MediaTracker class provides a media objects including images. Create an
instance of MediaTracker and call addImage() method which adds an image. The
method waitForAll() of this class loads the image which is added.
To wrap one or more data arrays
to store image data, we have used the class DataBuffer. The method getDataBuffer() retrieves the data
buffer. The method getSize() of DataBuffer class returns the size of all data
arrays. To return the requested element from the array, the method getElem(i)
is used. The
method setElem(j, buffer1.getElem(i)) sets the requested element in the array.
We have create two objects of class DataBuffer, i.e., buffer1 which
retrieves the the data buffer of bufferedImage1 and buffer2 retrieves the data
buffer of bufferedImage2. The two objects bufferedImage1 and bufferedImage2 of class
BufferedImage class acts as
a source image and the destination image respectively. The destination image is
the flipped image.
Here is the code of FlipImageExample.java
import java.awt.*;
import javax.swing.*;
import java.awt.event.*;
import java.awt.image.*;
public class FlipImageExample extends JFrame {
ShowPanel panel;
JButton button;
public FlipImageExample() {
super("Flip the image");
Container container = getContentPane();
panel = new ShowPanel();
container.add(panel);
Box box = Box.createHorizontalBox();
button = new JButton("Flip");
button.addActionListener(new ButtonListener());
box.add(Box.createHorizontalGlue());
box.add(button);
box.add(Box.createHorizontalGlue());
container.add(box, BorderLayout.NORTH);
addWindowListener(new WindowEventHandler());
setSize(350, 250);
setVisible(true);
}
class WindowEventHandler extends WindowAdapter {
public void windowClosing(WindowEvent e) {
System.exit(0);
}
}
public static void main(String arg[]) {
new FlipImageExample();
}
class ButtonListener implements ActionListener {
public void actionPerformed(ActionEvent e) {
if (!panel.flip) {
panel.flipImage();
panel.bufferedImage = panel.bufferedImage2;
panel.flip= true;
}
else {
panel.bufferedImage = panel.bufferedImage1;
panel.flip = false;
}
panel.repaint();
}
}
}
class ShowPanel extends JPanel {
BufferedImage bufferedImage, bufferedImage1, bufferedImage2;
boolean flip = false;
ShowPanel() {
setBackground(Color.white);
setSize(450, 400);
Image image = getToolkit().getImage("image4.jpg");
MediaTracker mediaTracker = new MediaTracker(this);
mediaTracker.addImage(image, 1);
try {
mediaTracker.waitForAll();
} catch (Exception e) {}
bufferedImage1 = new BufferedImage(image.getWidth(this),
image.getHeight(this),BufferedImage.TYPE_INT_ARGB);
Graphics2D g2d = bufferedImage1.createGraphics();
g2d.drawImage(image, 0, 0, this);
bufferedImage = bufferedImage1;
}
public void flipImage() {
bufferedImage2 = new BufferedImage(bufferedImage1.getWidth(),
bufferedImage1.getHeight(), bufferedImage1.getType());
DataBuffer buffer1 = bufferedImage1.getRaster().getDataBuffer();
DataBuffer buffer2 = bufferedImage2.getRaster().getDataBuffer();
for (int i = buffer1.getSize() - 1, j = 0; i >= 0; --i, j++) {
buffer2.setElem(j, buffer1.getElem(i));
}
}
public void paintComponent(Graphics g) {
Graphics2D g2D = (Graphics2D) g;
g2D.drawImage(bufferedImage, 0, 0, this);
}
}
|
Output will be displayed as:
On clicking the button, the image will get reverse.
]
Download Source Code