Displaying Hello using RMI
This Example describes the way to display Hello message using RMI. By RMI we mean Remote Method Invocation. RMI serves as a basic technique for supporting distributed objects in java. The steps involved in displaying message Hello are described below:-
Step1.Create a Remote interface named HelloInterface.java in the Directory.
HelloInterface.java:-
import java.rmi.*;
|
Step2.Create an Remote Class implementation for HelloWorld named Hello.java in the Directory.
Hello.java
import java.rmi.*;
|
Step3.Compile the above two Source file named HelloInterface.java and
Hello.java.
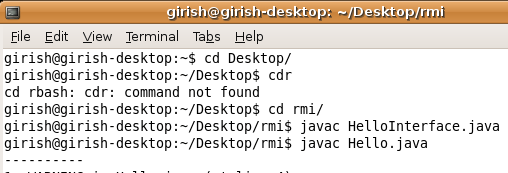
Step4.After compiling the above two classes type the following command i.e-"rmic Hello" in console just like displayed below.
Your Directory Structure will be like this.By running the "rmic Hello" command a new class will be created i.e "Hello_Stub.class" in the directory
Step5.Create Server application named HelloServer.java
HelloServer.java
import java.rmi.Naming;
|
Step6.Create Client application named HelloClient.java
HelloClient.java
import java.rmi.Naming;
|
Step6.Compile both of the files.
Step7.Type "rmicregistry" on commandprompt and press ENTER.
Step8.Type java HelloServer in commandprompt and press ENTER.The following message will be displayed on console.

Step9.Now,open another separate command terminal,and run the client application like shown in the figure given below:-
Step10. If the message similar to the above appears in figure comes means that you have implemented your RMI application.