In the following example, we have used the TreeSet collection, which is similar to TreeMap that stores its elements in a tree and maintain order of its elements based on their values.
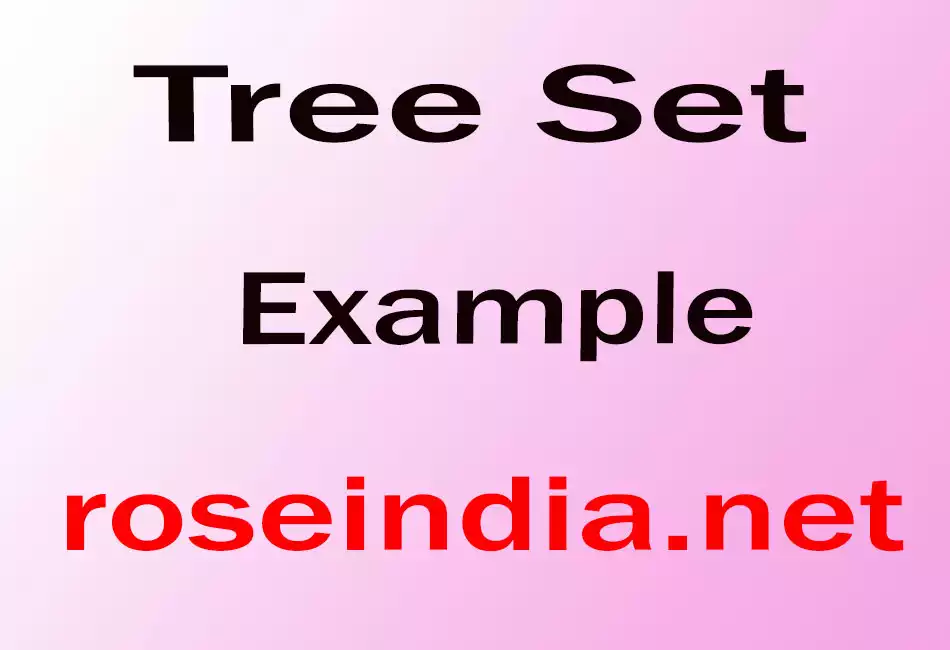
Tree Set Example

In the following example, we have used the TreeSet
collection, which is similar to TreeMap that stores its elements in a tree
and maintain order of its elements based on their values. To get the size of
TreeSet collection size() method is used. Our TreeSet collection contains
4 elements and the size of the TreeSet can be determine by calling size()
method.
Similarly, we have used first()
and last() to retrieve first and
last element present in the TreeSet. Program also shows the method to remove the
element and then display the remaining elements.
To remove all data from the TreeSet, use the clear()
method. To determine whether TreeSet is empty or not use isEmpty() method.
If the TreeSet is empty, it displays the message "Tree set is empty."
otherwise it displays the size of TreeSet.
Here is the code of program:
import java.util.*;
public class TreeSetExample{
public static void main(String[] args) {
System.out.println("Tree Set Example!\n");
TreeSet <Integer>tree = new TreeSet<Integer>();
tree.add(12);
tree.add(23);
tree.add(34);
tree.add(45);
Iterator iterator;
iterator = tree.iterator();
System.out.print("Tree set data: ");
//Displaying the Tree set data
while (iterator.hasNext()){
System.out.print(iterator.next() + " ");
}
System.out.println();
//Check impty or not
if (tree.isEmpty()){
System.out.print("Tree Set is empty.");
}
else{
System.out.println("Tree Set size: " + tree.size());
}
//Retrieve first data from tree set
System.out.println("First data: " + tree.first());
//Retrieve last data from tree set
System.out.println("Last data: " + tree.last());
if (tree.remove(30)){
System.out.println("Data is removed from tree set");
}
else{
System.out.println("Data doesn't exist!");
}
System.out.print("Now the tree set contain: ");
iterator = tree.iterator();
//Displaying the Tree set data
while (iterator.hasNext()){
System.out.print(iterator.next() + " ");
}
System.out.println();
System.out.println("Now the size of tree set: " + tree.size());
//Remove all
tree.clear();
if (tree.isEmpty()){
System.out.print("Tree Set is empty.");
}
else{
System.out.println("Tree Set size: " + tree.size());
}
}
}
|
Download this example.
Output of this program:
C:\vinod\collection>javac TreeSetExample.java
C:\vinod\collection>java TreeSetExample
Tree Set Example!
Tree set data: 12 23 34 45
Tree Set size: 4
First data: 12
Last data: 45
Data doesn't exist!
Now the tree set contain: 12 23 34 45
Now the size of tree set: 4
Tree Set is empty.
C:\vinod\collection> |